API Change Notice:
The "Raptor App API" was renamed to "Raptor App Legacy API" and the endpoint was changed to https://app-legacy.raptormaps.com instead of https://app.raptormaps.com on April 4, 2023.
Note that https://api.raptormaps.com is the latest API as of June 2021. "Raptor App Legacy API" will continue to be deprecated.
Third-party data ingestion still remains at [https://app-legacy.raptormaps.com].
Introduction
Welcome to Raptor Maps' API documentation. We provide this API to increase data access for our customers. There is a Postman collection available as a supplement to this documentation.
Authentication
Raptor Maps is using a secure system for permanent API credentials that allow users to receive temporary API tokens. This now means a 2 step process to get your API token:
- Creating API credentials for your user in the Raptor App at the My Profile page
- Sending a request with these credentials to our Auth API that will return an expiring API Key (AKA Access Token)
Let's walk through these steps in detail to get you started with the Raptor Maps API!
Creating API Credentials
If you have existing credentials, you should see your credentials loaded shortly after navigating to the Profile Page and can skip this section.
- Navigate to the My Profile page of the Raptor App.
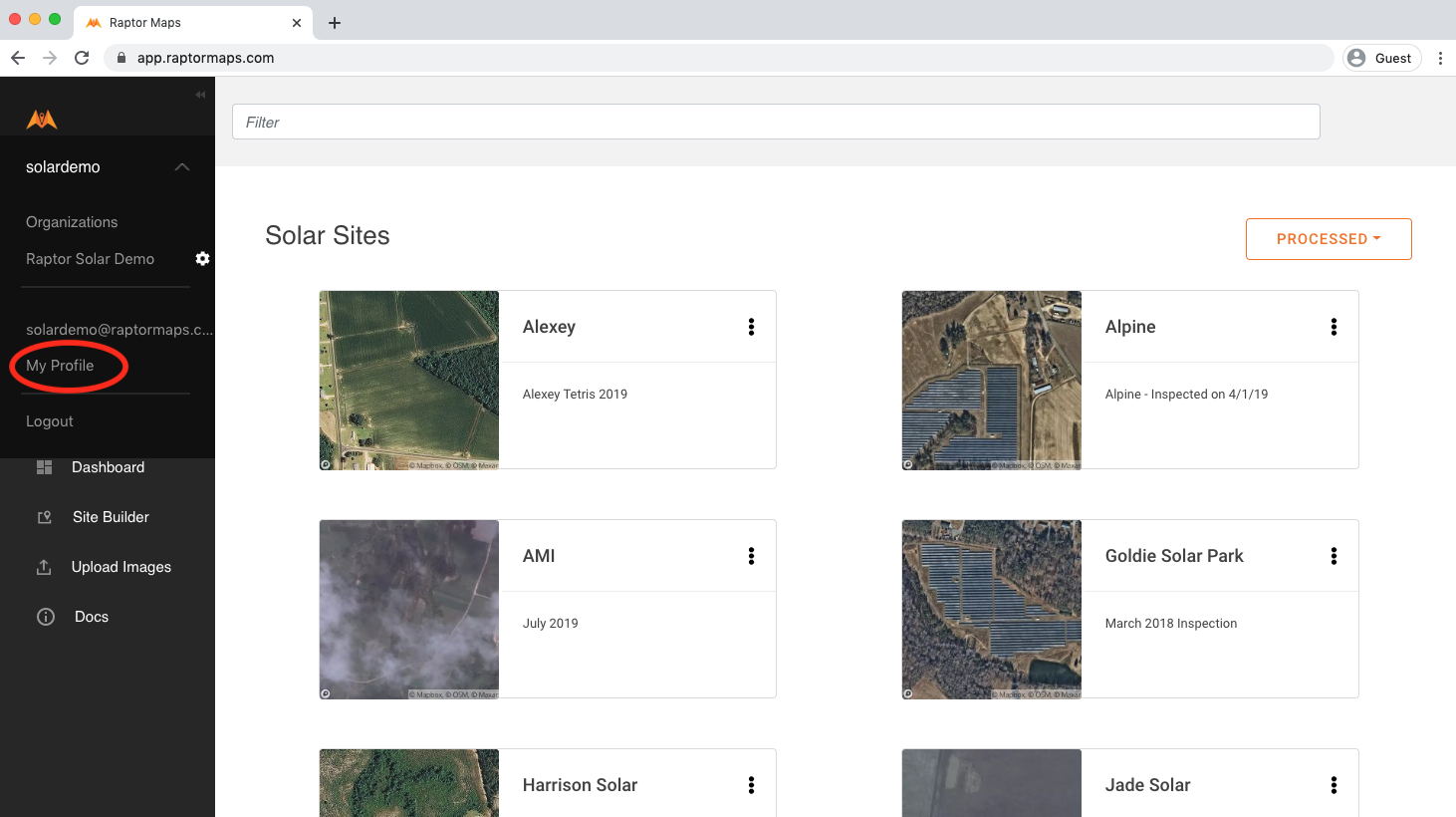
Navigate to My Profile
- New API users without existing credentials should see the Create API Credentials button under the API Credentials section. Click this to create your credentials!
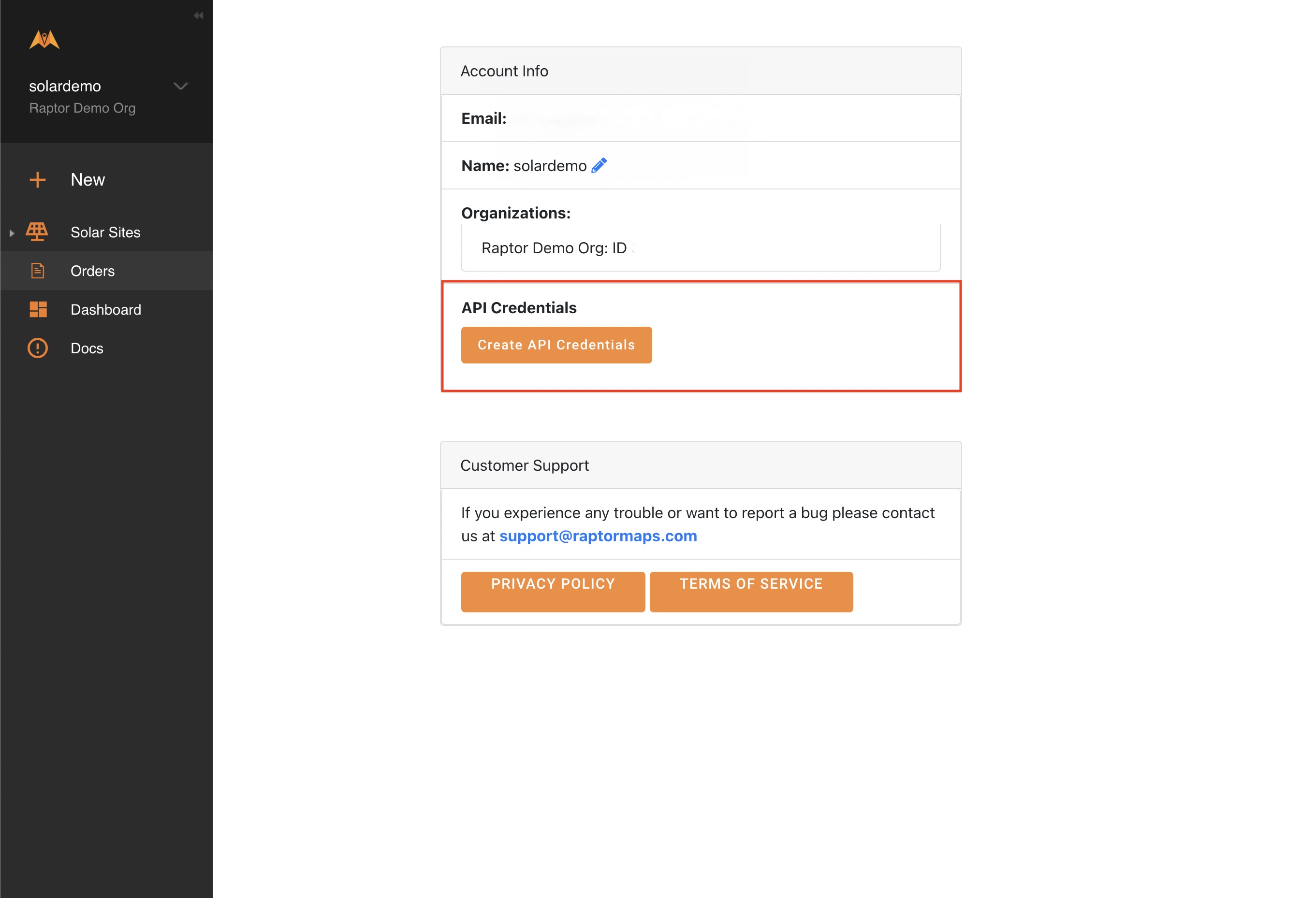
Create API Credentials
- Your credentials will appear on screen once created!
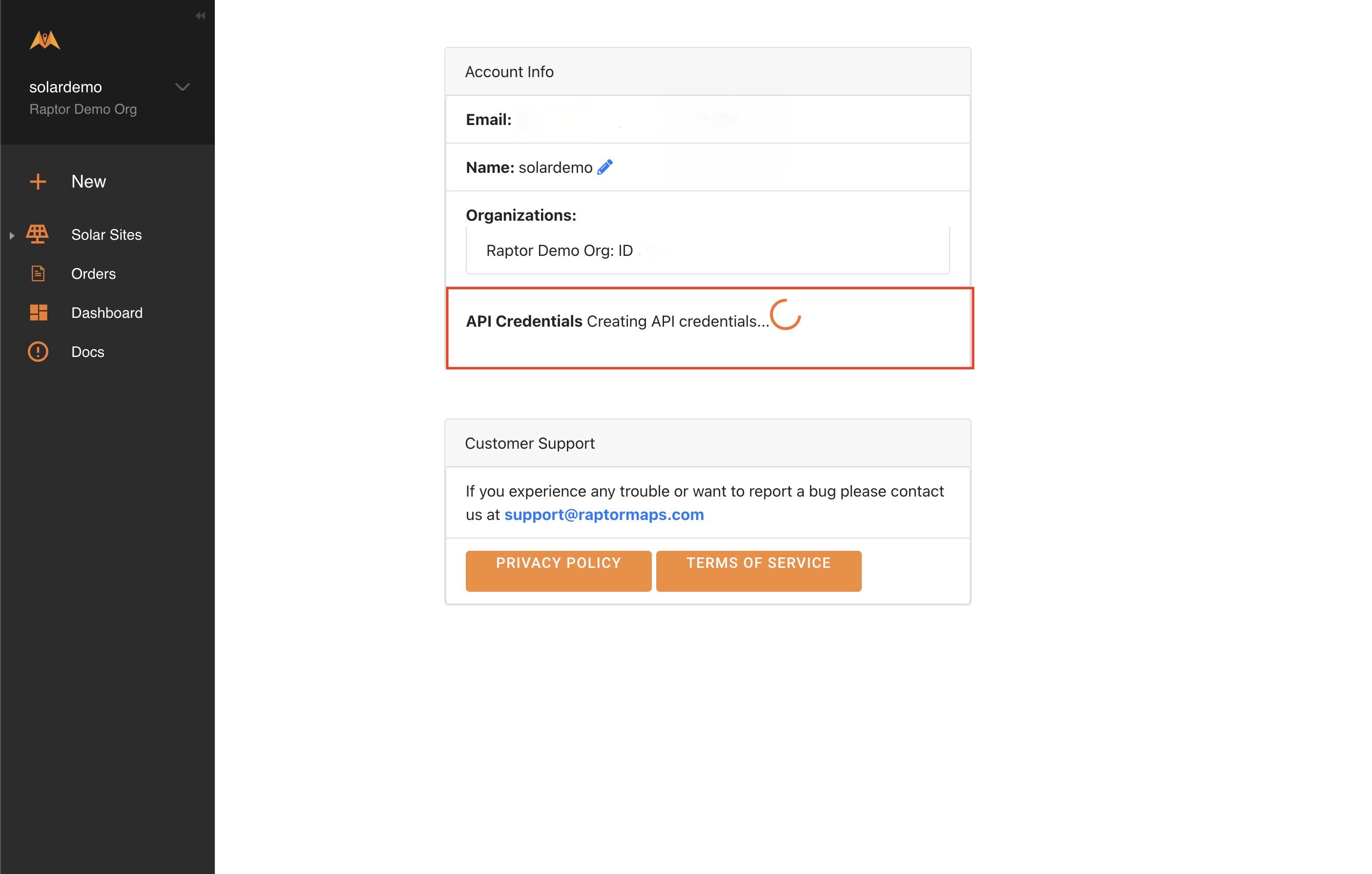
Creating API Credentials In Progress
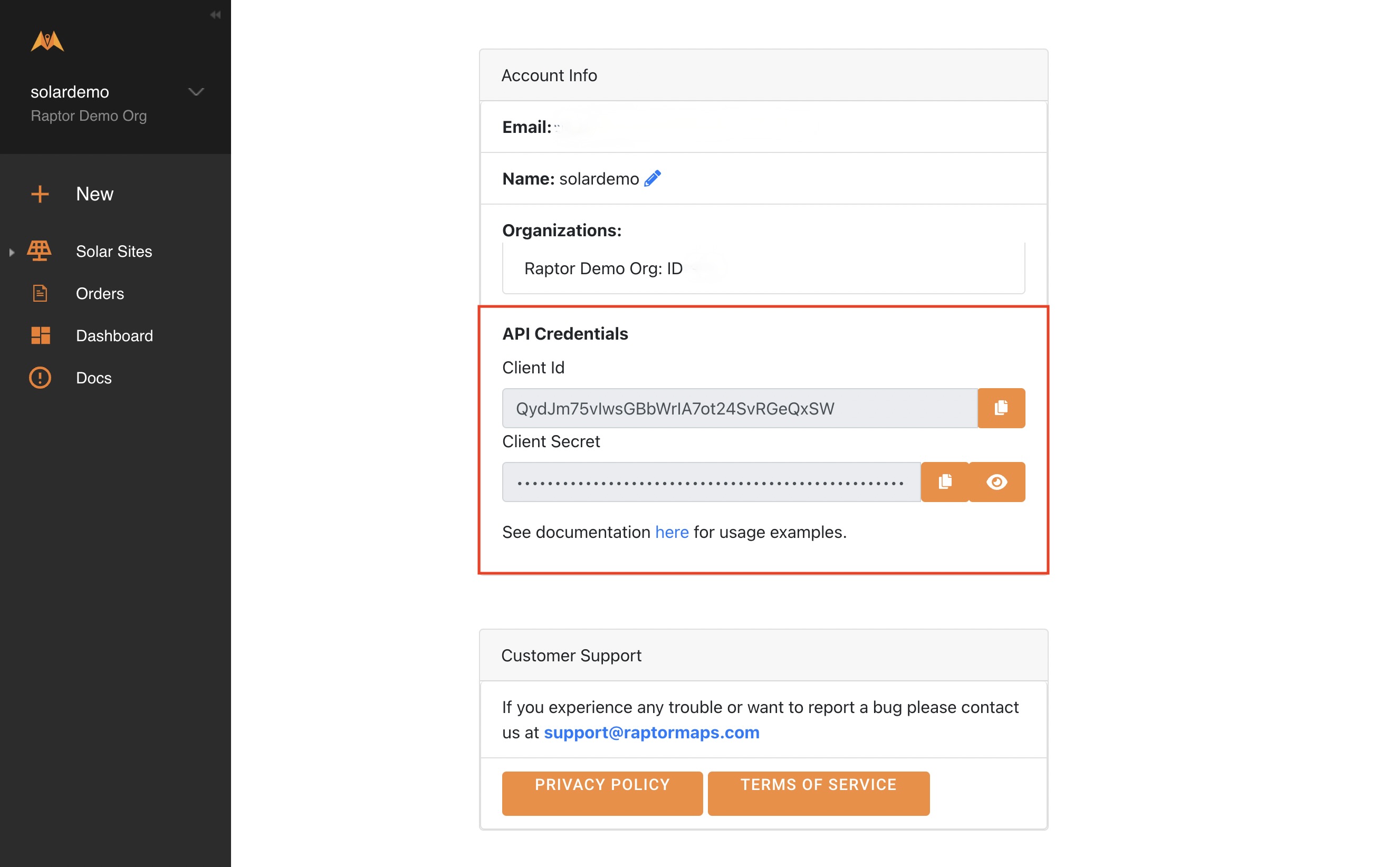
API Credentials successfully created!
IMPORTANT - Make sure you never give your Client Id & Client Secret combination to anyone you do not trust - anyone with this information will be able to authenticate against our application as your user.
If you suspect your API credentials are being misused by unauthorized parties, Raptor Maps can revoke access to allow you to create new API credentials.
Using API Credentials
Setting Up Your Environment
Once you have your API credentials, you may want to save these values to your system's environment variables. We've chosen to save them as:
- RM_API_CLIENT_ID
- RM_API_CLIENT_SECRET
These values will be used to request & receive an actual API token you can use to hit our API endpoints.
Getting an Org ID
org_id
is a commonly required query parameter to our API calls for authorization to view specific data.
To retrieve an ORG_ID value, visit My Profile and look under the Organizations section. You may have multiple ORG_ID values, so make sure you are using the correct one for the data you are requesting.
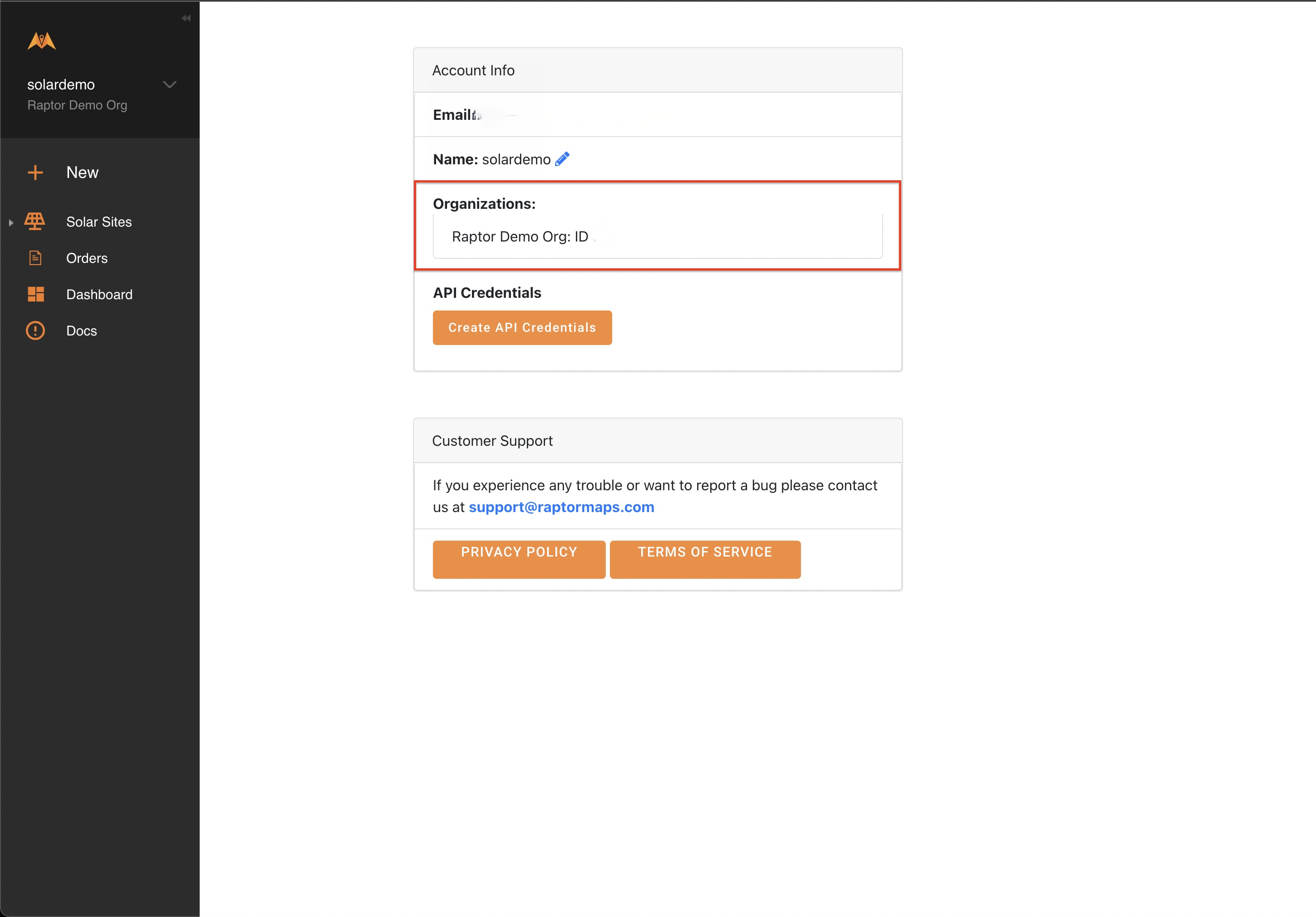
Find your Org IDs here
Using The Raptor Maps API
Raptor Maps provides an API endpoint that returns an access token to you.
Visit the Get API Access Token page for detailed documentation.
You can now test getting a token directly from that page, for your convenience.
This endpoint can be used for programmatic scripts, one-off access using a client like Postman or even cURL, and more.
For one-off access, remember that the API token will expire in 30 days. You can simply use the endpoint again to retrieve a new access token if you receive a token_expired
error when accessing our API.
Sample Python Script
The following example script written in python that retrieves a token from the endpoint, and then requests solar farm data from the API, using the RM_API_CLIENT_ID and RM_API_CLIENT_SECRET environment variables we set up in the previous Setting Up Your Environment section.
import requests
import os
import json
# Otherwise, retrieve one using our API credentials
client_id = os.environ['RM_API_CLIENT_ID']
client_secret = os.environ['RM_API_CLIENT_SECRET']
token_endpoint = 'https://api.raptormaps.com/oauth/token'
token_endpoint_headers = {
'content-type': 'application/json'
}
token_endpoint_body = {
'client_id': client_id,
'client_secret': client_secret,
'audience': 'api://customer-api'
}
token_response = requests.post(
token_endpoint,
headers=token_endpoint_headers,
data=json.dumps(token_endpoint_body))
response_data = token_response.json()
api_access_token = response_data.get('access_token')
print(f'api access token: {api_access_token}')
BASE_URL = 'https://api.raptormaps.com'
ORG_ID = 101 # Update this to your desired org ID
SOLAR_FARM_ID = 100 # Update this to your desired solar farm ID
# Load a specific solar farm's metadata
# Specify API endpoint using your SOLAR_FARM_ID
endpoint = f"{BASE_URL}/solar_farms/{SOLAR_FARM_ID}"
# Specify request headers
headers = {
'content-type': 'application/json',
'Authorization': f'Bearer {api_access_token}'
}
# Specify request parameters
query_params = {
'org_id' : ORG_ID
}
# Make HTTP GET request
r = requests.get(endpoint, headers=headers, params=query_params)
# Parse response
data = r.json()
print(f'Solar Farm Data: {data}')
print('Request Complete!')
At this point you should be authenticated into the Raptor App. Enjoy.